Eclipse is one of the leading IDEs (development environments) for Java developers. And it’s the basis of IBM RAD Developer, Springsource STS, and other tools. One reason I like Eclipse is that it is so thoughtfully crafted for optimal developer productivity. There are a jillion shortcuts that - if you take the time to learn them - will save you many hours of boring typing, giving you far more time to write tests or play Pong or whatever.
In particular, Eclipse is optimized for keyboard shortcuts. Think about what happens when you pick something from a menu:
-
Your brain realizes you have to pick your hand up; your muscles do so.
-
Your brain realizes you have to move your hand over to the mouse: your muscles make it so.
-
The same pattern repeats as you grasp the mouse;
-
move your eyes to the menu;
-
remember which mouse button to click, and click it;
-
slide the mouse down to the menu you want;
-
click the mouse button on the menu item;
-
repeat the first four steps in reverse order.
PSA: You just wasted one heck of a lot of neural energy. Ever wonder why you feel tired at the end of the day (or sooner)?
Instead, you can just tap a key where your fingers already are, or even "just press enter." Boom! I fixed it for you.
So without further ado, here are some of my favorites. There is a a built-in list of the keyboard shortcuts, vit CTRL/Shift/L. These are, of courese, in addition to the "common" keyboard shortcuts for cut/copy/paste that work almost everywhere.
Mac users: some of the places I use CTRL, you’ll use COMMAND instead. For example, on a Mac, the list of keyboard shortcuts is COMMAND/Shift/L. But not all of them work that way; see the online docs for the ones that don’t "just work".
Ian’s Rule
Immodest to name it after myself, but here goes:
Never type more than 3 or 4 letters of any identifier, except when creating it."
Eclipse knows everything there is to know about your project, all your code, and all the libraries that are part of the app. You never need to type those long names! See the first shortcut below to see how to avoid breaking this important rule.
Finish the job with Ctrl/space
When you type the first few letters of a name… nothing happens. But when you activate that with CTRL/Space, Eclipse springs to life with a flurry of suggestions, which are, all the possible completions, with the most likely completions first (see for example After Typing "Sys"+CTRL/Space for System.out). If the first one is right, just hit Enter with your pinky finger and it’s done! If the second or third is right, just pinky the Down Arrow a few times and press Enter. If the one you want is way down, whoa! Still don’t use the mouse. Just type one or two more letters until you get the one you want at the top (I know, I said never type more than 3 or 4 letters, but this is an exception).
Big savings in neural energy (and mouse motion), and time!

BTW, I’ve seen people working on a line like
SomeLongTypeName myVar = new
at which point they will laboriously select the type name with the mouse, then right click and select Copy from the menu, then right-click after the '=', select Paste, and type '();'. Wow! All they needed to do was to type CTRL/SPACE after typing the 'new', and Eclipse will provide the same type name for the assignment as is present in the declaration. Eclipse knows! Use its knowledge.
The Dot-Pause That Refreshes
When you have typed the name of a class or instance, and type the "." to separate it from a method or field, just stop! After a split second, Eclipse will pop up a list of suggestions. Proceed as you did with CTRL/Space above and you’ll have that long name filled in by Eclipse in no time flat.
Seeing Red? One Quick Fix after another, under Control
CTRL/1 is your friend when you and Eclipse see red. Eclipse spots all syntax errors including undefined references; most common errors are recognized by the "Quick Fix" mechanism and and be repaired in a jiffy with CTRL/1. As with CTRL/Space, there will often be multiple choices, which you can get to with the arrow key and press Enter when you reach the correct fix. If you don’t want to use Quick Fix, hit Escape to dismiss it.
Sysout And Other Templates
Take the pledge now! "My name is (put your name here) and I will never, ever type out…
System.out.println(foo);
again." Just type sysout (or even syso) and then - guess what? - CTRL/Space. Bingo - typing completed for you. Just finish up the expression to be printed.
There are numerous other templates; look in the Preferences window, under Java→Editor→Templates for a full list. If you click on one, you’ll see what it expands to in the Preview window. Macros use ${…} substitution syntax.
DIY Templates
In fact, while you’re on the Templates page, why not bake your own, for something you personally (or your team) use a lot. Suppose we use a lot of Logger objects, which we get in each class with a LogFactory. Click New, then fill in Logger for the name, some text like "Get a logger for this class" for the Description, and for the Preview, put something like this, depending on which of the (too many) Java Logging APIs you are using:
static Logger logger = LogFactory.getLogger(${enclosing_type}.class);
The result should look something like this, before you press OK:
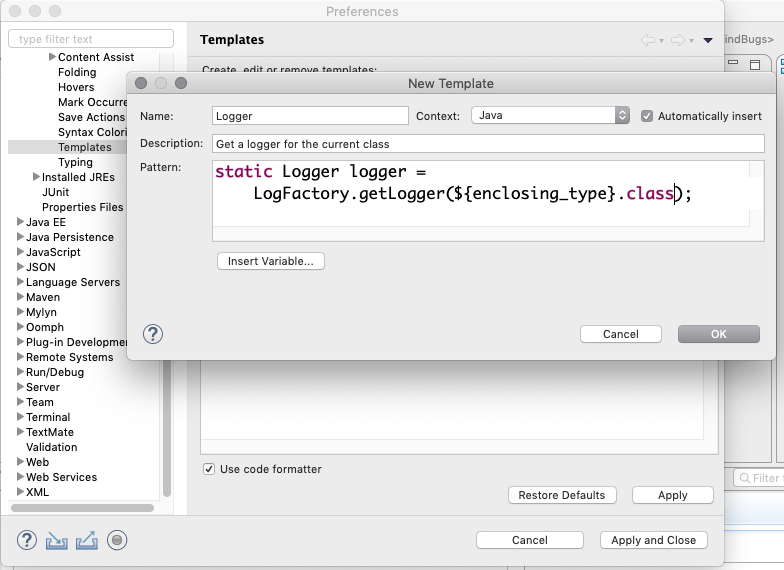
Then to use it, just type log<CTRL/SPACE>, choose Logger (find the name in blue with your description beside it), and watch the full statment appear! If you made a mistake, no worries, just go back into the same Preferences pane as above, select your custom template, and click Edit. When done click Apply.
To share it with your team, go back into the Preferences pane, select your new template (or more than one), click Export, and save it where others can get it. They just use Import in the same place.
Let the unset be ungotten
Never, ever type out public void setName(String name) { this.name = name; }
.
Nor, I might add, the corresponding getName()
. Just create the field
String name;
and let Eclipse do the work! Source→Generate Getters and Setters
,
pick the fields you want getters and/or setters for (often just
Select Getters
(for immutable objects, else Select All
), and click OK
. Presto! Job done.
Similarly for hashCode()
and equals()
, constructors using fields,
overrides, and even toString()
.
Note that if you don’t want to bulk up your code with these "boilerplate" methods, Lombok (https://projectlombok.org/) will generate them dynamically.
Better yet, Java 16+ record
types will also automate generation of these methods.
In fact, a record
type will replace your immutable (read-only) data classes; you just write
the class name and the list of properties
(their one annoyance is that the getters don’t have get
at the front, just the property name;
this is simpler, but a step back from a decades-long tradtion in Java).
Direct Us Not Into Temptation
To open a file when you know its class name but don’t want to bother rummaging around in various directories to find it, just do CTRL/SHIFT/R (R for Resource) or T (for Type). Enter the first three characters in the name box that pops up, and follow the by-now usual rules for narrowing down the selection until you can press Enter.
Scrolling, Scrolling, On The Endless Sea
Java source files can be hundreds or even thousands of lines long. Scrolling through them to find errors is a big time sink.
First, if you know the line number you want to reach, CTRL/L will let you jump directly to that line.
Look at the right margin of the editor window. If you see a little blue box, that is a TODO item (marked by TODO or XXX in the code). Just click on the little blue box and Eclipse will jump you there. If you see a little red box, that’s an error. Again, clicking on the red box will take you right to the line with the error. Ditto for yellow, which indicates a warning.
BTW, You should eliminate all warnings as you go, from the beginning of your project. Some warnings are useless and some warn of terrible times ahead for your code. It takes some judgement to know which is which, but at least make a stab at eliminating the warnings.
And speaking of scrolling, what about when you see a stack trace in the console, or an error in JUnit output (you do use JUnit religiously, don’t you?). When you see lines in either place that are underlined, just click on them, and you will miraculously jump to that part of the code in an editor window, even if the file wasn’t already open. Really neat!
There is no try/catch, there is only do
Don’t sit around typing
try { ... } catch (ThisException | ThatException | TheOtherException e) {...}
(Unless it’s some form of job security by slowing down your typing). Just type the code that throws an exception, which will turn red, and use Quick Fix as above, and choose Surround with Multi-Catch. Job done! Well, almost. You have to use some intelligence to provide decent error handling, but that’s gist for another document.
There’s also "Add to surrounding multi-catch" if you add additional API calls with additional checked exception(s) inside an existing try.
No Comment?
To comment out lines of code, select them and press CTRL-Slash. To use a block comment, Shift/CTRL-Slash. It’s a toggle, so selecting commented-out lines of code and typing CTRL-Slash will uncomment them.
Clean That Mess with Ctrl/Shift/O
Hate that ring of ugly imports that grew like topsy and is in random order? Clean it up with CTRL/Shift/O for Organize Imports.
Note also that if you follow Ian’s Rule above, you rarely have to type import statements, because when Eclipse completes a type name that hasn’t yet been imported, it will add the import statement for free, and try to keep it in organized order if it can.
Never Say Ever Again
Eclipse is full of confirmation dialogs. The majority of these have a "Don’t Ask Again" type checkbox, with various wordings. Almost all of those are good to check the first time you see them, assuming that you know what you’re doing and don’t want to be bothered confirming that you meant what you said, every time.
The most important one is "Always save changed files before running", which you’ll see every single time you run a program in Eclipse, until you notice the little check box and check it when running an application.
Many Devs Open Many Repos with Team Project Set
Suppose you need everyone on your team to open the same set of GIT repositories for a new project. You could give them verbal instructions, but that’s error-prone. You could give them written (aka emailed) instructions to copy-and-paste the URLs, but that’s tedious for all concerned. Instead, you want to send them a PSF file. PSF stands for Project Set File, an XML format created by & for Eclipse for this purpose. Here’s how it works:
-
Import the projects from git into your own Eclipse workspace.
-
Go to File→Export…, then choose Team→Team Project Set.
-
Select all the projects you want included. Click Next.
-
Choose where to put the PSF, either inside a project in the workspace (convenient if you have one project already shared through your SCM), in the disk filesystem, or on an intranet web server.
-
Click Finish.
-
Here is what my PSF looks like, for some projects I often load onto temporary computers: EclipseProjectSet.psf
-
If you saved it to a file, put it on a shared network drive, or on a web server, or a USB memory device.
-
Now you just have to tell people to use File-Import, Team→Team Project Set, and then specify the URL or path to your PSF, and click Finish.
-
Boom - they’re done. Well, almost. Some projects will show Reds (errors) for several minutes as dependencies are downloaded in the background.
But Wait: There’s More!
I can not claim to have a complete list of the shortcuts baked into Eclipse. I can say that they have saved me oodles of time, and I’d like them to work for you. If you’ve read this whole article, check out this free download of my Eclipse Shortcuts Poster. And while you’re at it, please buy a copy of my fine Java Cookbook.